Документація SMS
У SMSmobileAPI ми прагнемо зробити наш API максимально сумісним із будь-якою архітектурою чи платформою.
Незалежно від того, чи використовуєте ви REST, SOAP, cURL або різні мови програмування, як-от Python, JavaScript, PHP тощо, наш API створено для бездоганної інтеграції у ваші проекти.
У нас також є готові до використання плагіни для рішень електронної комерції та програмного забезпечення. Натисніть тут, щоб дізнатися про них.
.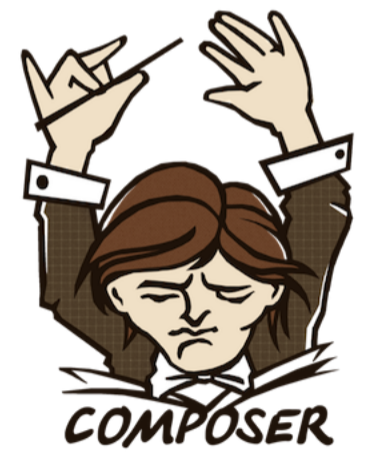
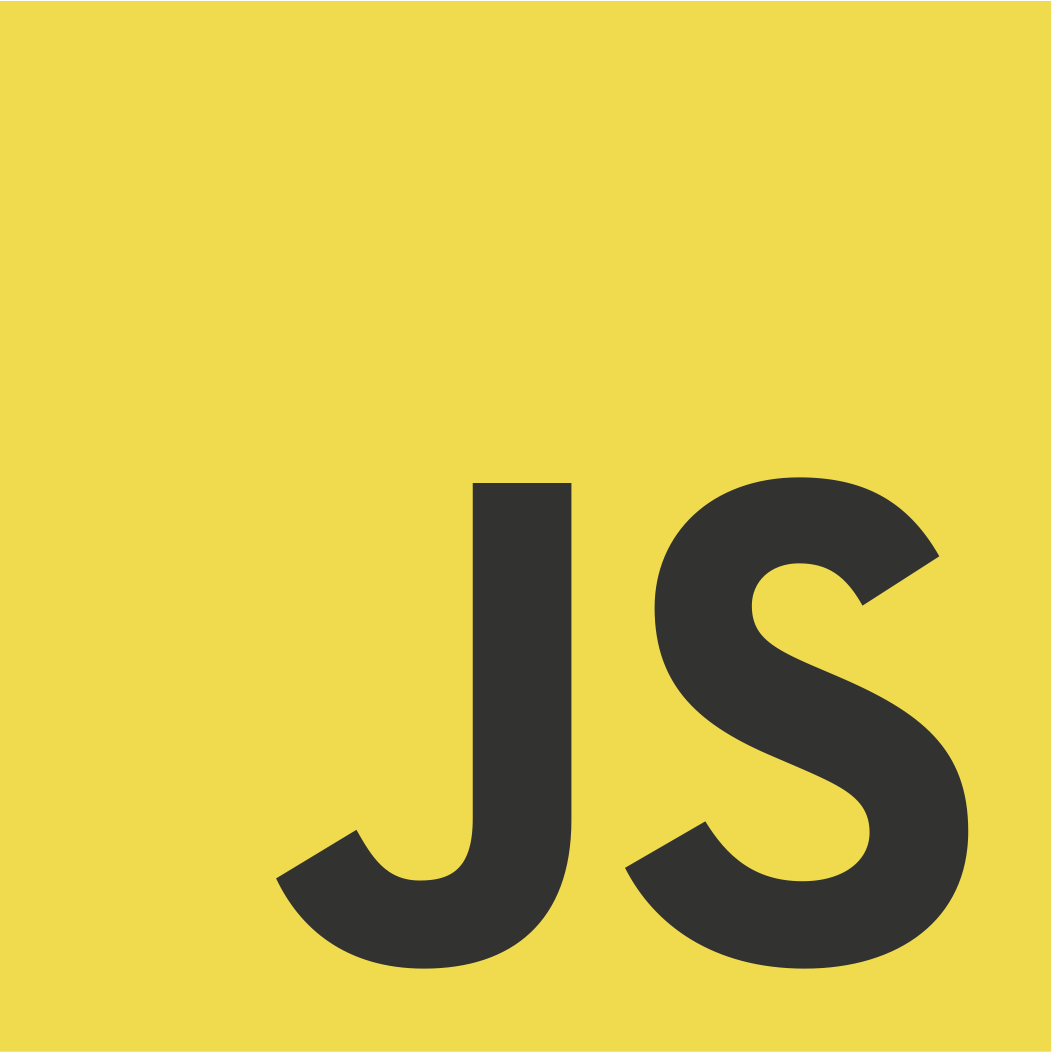
Надіслати SMSПовторно надіслати невідправлене SMSLOG SMS надісланоОтримайте SMSПозначити SMS-повідомлення як прочитаніВидалити SMSОтримано SMS - вебхукАутентифікація |
Надіслати SMSЦя кінцева точка дозволяє надсилати SMS зі свого мобільного телефону.Кінцева точка:ОТРИМАТИ ПОСТ https://api.smsmobileapi.com/sendsms/
Параметри:
приклад:
ОТРИМАТИ https://api.smsmobileapi.com/sendsms?apikey=YOUR_API_KEY&recipients=+1234567890&message=HelloWorld
Повторно надіслати невідправлене SMSЦя кінцева точка API використовується для повторного надсилання невідправленого SMS (лише якщо повідомлення має статус помилки) Кінцева точка:Параметр:
приклад:
ОТРИМАТИ https://api.smsmobileapi.com/resend/?apikey=YOUR_API_KEY&guid=GUID_OF_THE_MESSAGE
Журнал SMS, надісланих з APIЦя кінцева точка API використовується для отримання журналу SMS-повідомлень, надісланих через API. Кінцева точка:ОТРИМАТИ https://api.smsmobileapi.com/log/sent/sms/
Параметр:
приклад:SMS отриманоЦя кінцева точка API використовується для отримання SMS-повідомлень, отриманих на смартфон. Кінцева точка:ОТРИМАТИ https://api.smsmobileapi.com/getsms/
Параметр:
приклад:Позначити отримані SMS-повідомлення як прочитані
Ця кінцева точка API використовується для позначення одного або кількох отриманих SMS-повідомлень як прочитаних лише у статусі API. Кінцева точка:ОТРИМАТИ https://api.smsmobileapi.com/getsms/set-read/
Параметр:
приклад:
ОТРИМАТИ https://api.smsmobileapi.com/getsms/set-read/?apikey=YOUR_APIKEY&guid_message=GUID_MESSAGE
Видалити SMSЦя кінцева точка API використовується для видалення SMS-повідомлень із журналу сервера SMS Mobile API Кінцева точка:ОТРИМАТИ https://api.smsmobileapi.com/deletesms/
Параметр:
приклад:
ОТРИМАТИ https://api.smsmobileapi.com/deletesms/?apikey=ВАШ_API_KEY
Примітка. Видалені SMS – це лише ті, що зберігаються в журналах вашого облікового запису мобільного додатку. СМС на самому мобільному пристрої видалятися не будуть, так як ми не маємо до них доступу. Вебхук – SMS отриманоЦя система webhook надсилає запит POST на налаштовану URL-адресу кожного разу, коли надходить SMS. Система забезпечує оновлення в реальному часі, доставляючи деталі SMS на вказану URL-адресу веб-хуку. Як налаштувати вебхук на інформаційній панеліВиконайте наведені нижче дії, щоб налаштувати URL-адресу веб-хуку на інформаційній панелі:
Корисне навантаження вебхукуКоли надходить SMS, система надсилає таке корисне навантаження JSON на вашу URL-адресу веб-хука:
Поля корисного навантаження:
Приклад кінцевої точки WebhookВаш сервер має бути готовий обробляти вхідні запити POST. Нижче наведено приклад сценарію PHP для обробки корисного навантаження вебхуку:
Тестування WebhookЩоб перевірити конфігурацію вебхуку, скористайтеся такими інструментами, як: Ці інструменти дозволяють перевіряти корисне навантаження, надіслане системою, і виправляти будь-які проблеми з налаштуванням веб-хуку. Усунення несправностей
АутентифікаціяAPI SMSMobile підтримує два методи автентифікації: за допомогою простого ключа API або протоколу OAuth2 з ідентифікатором клієнта та секретом клієнта. 1. Аутентифікація ключа APIДля цього методу потрібен ключ API, який можна включити як параметр у запит GET або POST. Це простий спосіб автентифікації ваших запитів API. 2. Автентифікація OAuth2OAuth2 забезпечує більш безпечний і масштабований метод автентифікації. Завантажте мобільний додаток зараз або отримати доступ до панелі керування. Отримання маркера доступуЩоб отримати маркер доступу, надішліть запит POST кінцевій точці маркера з ідентифікатором клієнта та секретом клієнта.
curl -X POST https://api.smsmobileapi.com/oauth2/token \
Використання маркера доступу:Отримавши маркер доступу, додайте його до Авторизація заголовок ваших запитів API:
curl -X POST https://api.smsmobileapi.com/sendsms \
Який метод слід використовувати?- Використовуйте Аутентифікація ключа API для швидкої та простої інтеграції. - Використовуйте Автентифікація OAuth2 для підвищення безпеки та масштабованості ваших інтеграцій. Для отримання додаткової інформації зверніться до повної документації. |
Ви можете надсилати повідомлення WhatsApp лише через наш API. Натисніть тут, щоб дізнатися більше.
Якщо у вас виникли запитання, пропозиції чи потрібна допомога, наша команда готова допомогти.
Не соромтеся звертатися до нас за адресою api@smsmobileapi.com
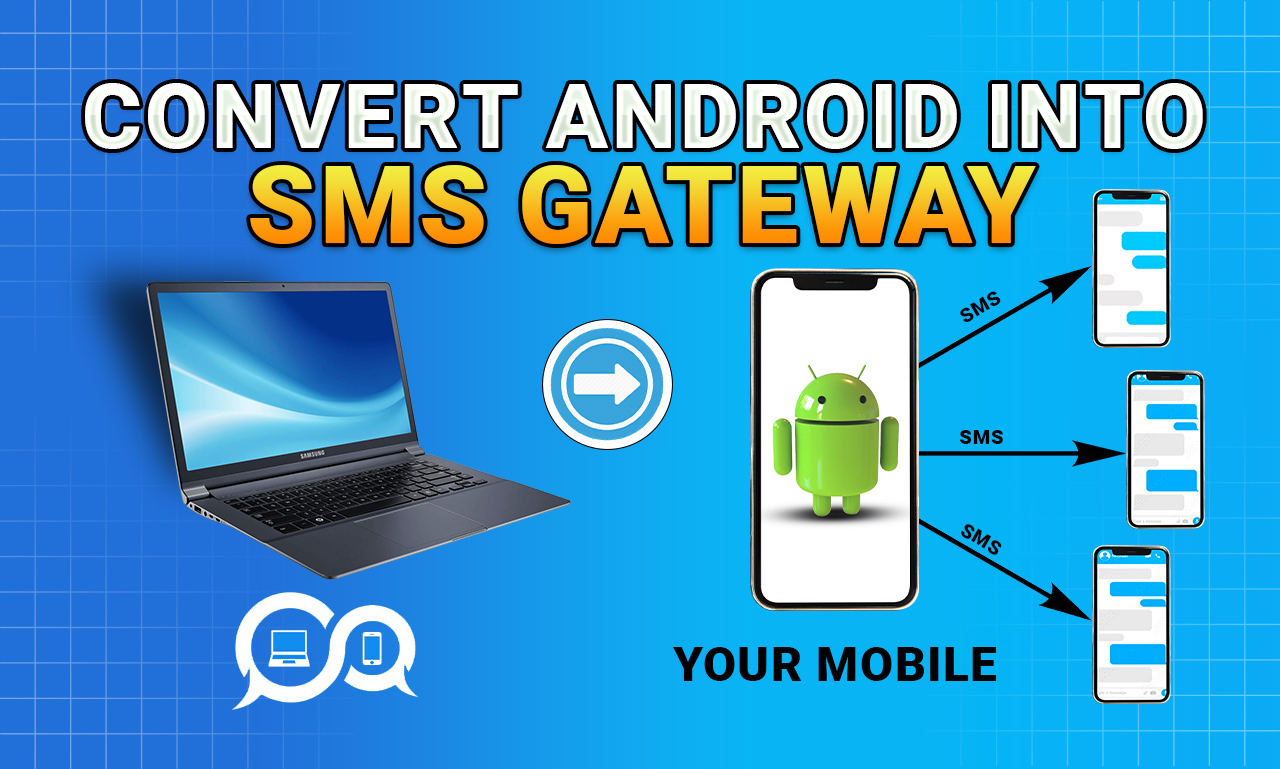
Ця проблема виникає через процес, який намагається запуститися у фоновому режимі, коли програма не запускається активно. Однак через заходи з оптимізації акумулятора Android, які відрізняються в різних версіях, цей фоновий процес може розпочатися неправильно. Оптимізація батареї Android призначена для обмеження фонової активності програм для збереження часу роботи батареї, що може ненавмисно вплинути на програми, які потребують фонових процесів для належного функціонування.
Щоб вирішити цю проблему, користувачі можуть вручну налаштувати параметри свого Android, щоб дозволити SmsMobileApi використовувати ресурси без обмежень.
Це передбачає коригування параметрів оптимізації заряду батареї для певної програми, по суті, вказуючи Android, що SmsMobileApi має дозвіл працювати у фоновому режимі та використовувати ресурси за потреби. Завдяки цьому програма повинна мати можливість автоматично надсилати й отримувати SMS-повідомлення, навіть якщо вона не є активною програмою на передньому плані. Це налаштування гарантує, що необхідний фоновий процес може працювати безперервно, обходячи функції оптимізації батареї, які інакше могли б перешкодити його належному виконанню.
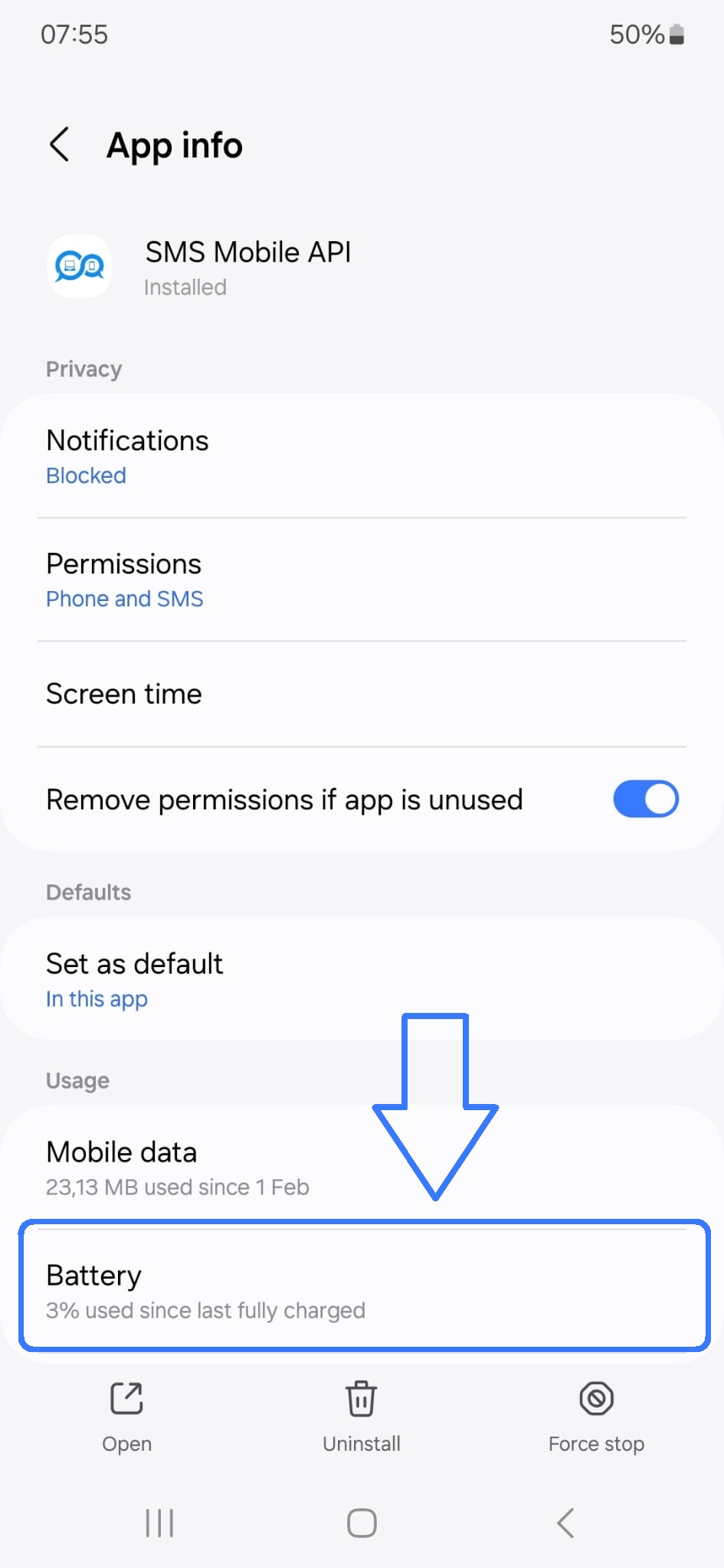
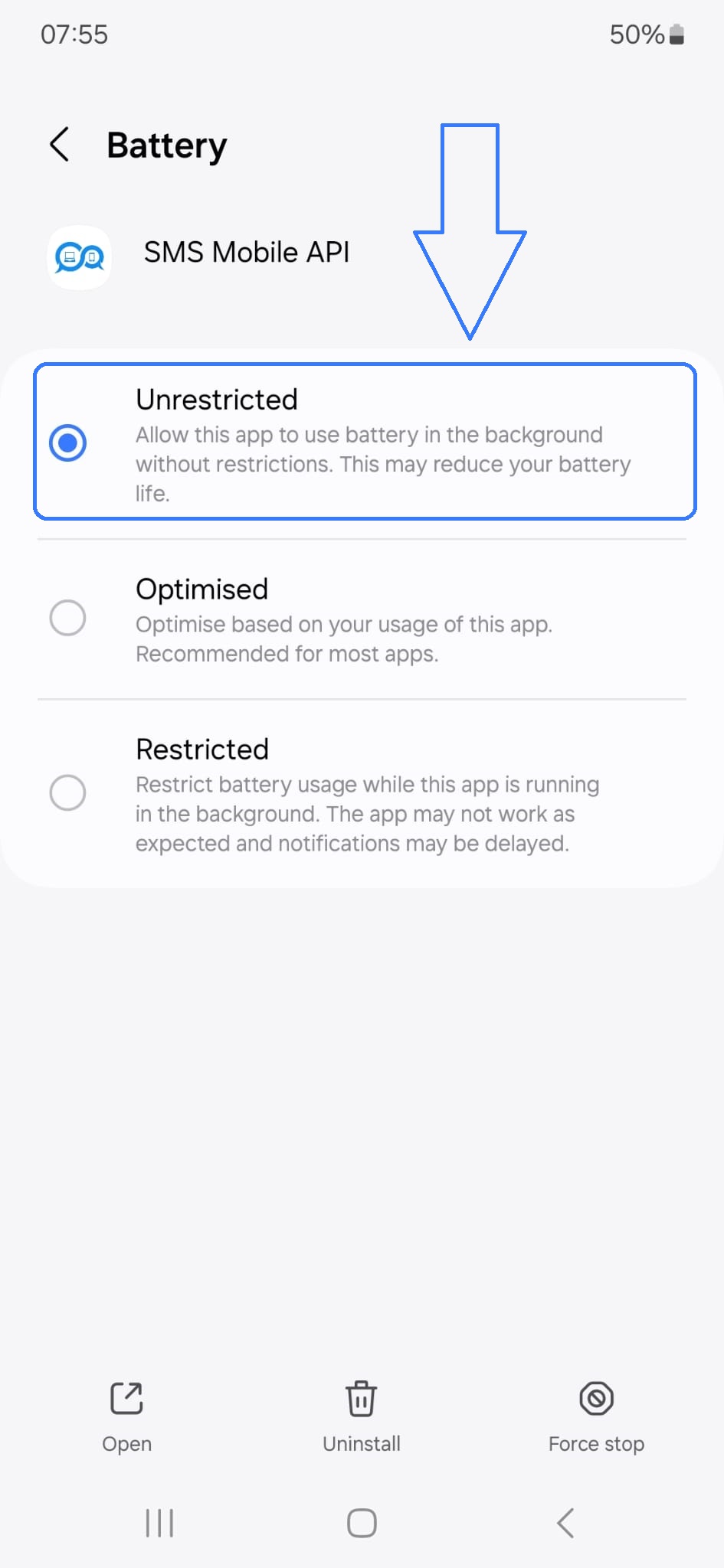
FAQ для розробника
Почніть роботу з SMSmobileAPI вже сьогодні!
Відчуйте свободу та гнучкість керування своїми службами SMS у дорозі. Завантажте наш мобільний додаток зараз і зробіть перший крок до повної інтеграції SMS.
Завантажте додаток