WhatsApp-documentatie
Deze documentatie is specifiek voor het verzenden en ontvangen van WhatsApp-berichten.Klik hier voor meer informatie over alle functies die onze WhatsApp-integratie biedt.
Bij SMSmobileAPI streven we ernaar om onze API zo compatibel mogelijk te maken met elke architectuur en elk platform.
Of u nu REST, SOAP, cURL of verschillende programmeertalen zoals Python, JavaScript, PHP en meer gebruikt, onze API is ontworpen om naadloos te integreren in uw projecten.
Wij hebben ook kant-en-klare plugins voor e-commerce oplossingen en software. Klik hier om ze te ontdekken.
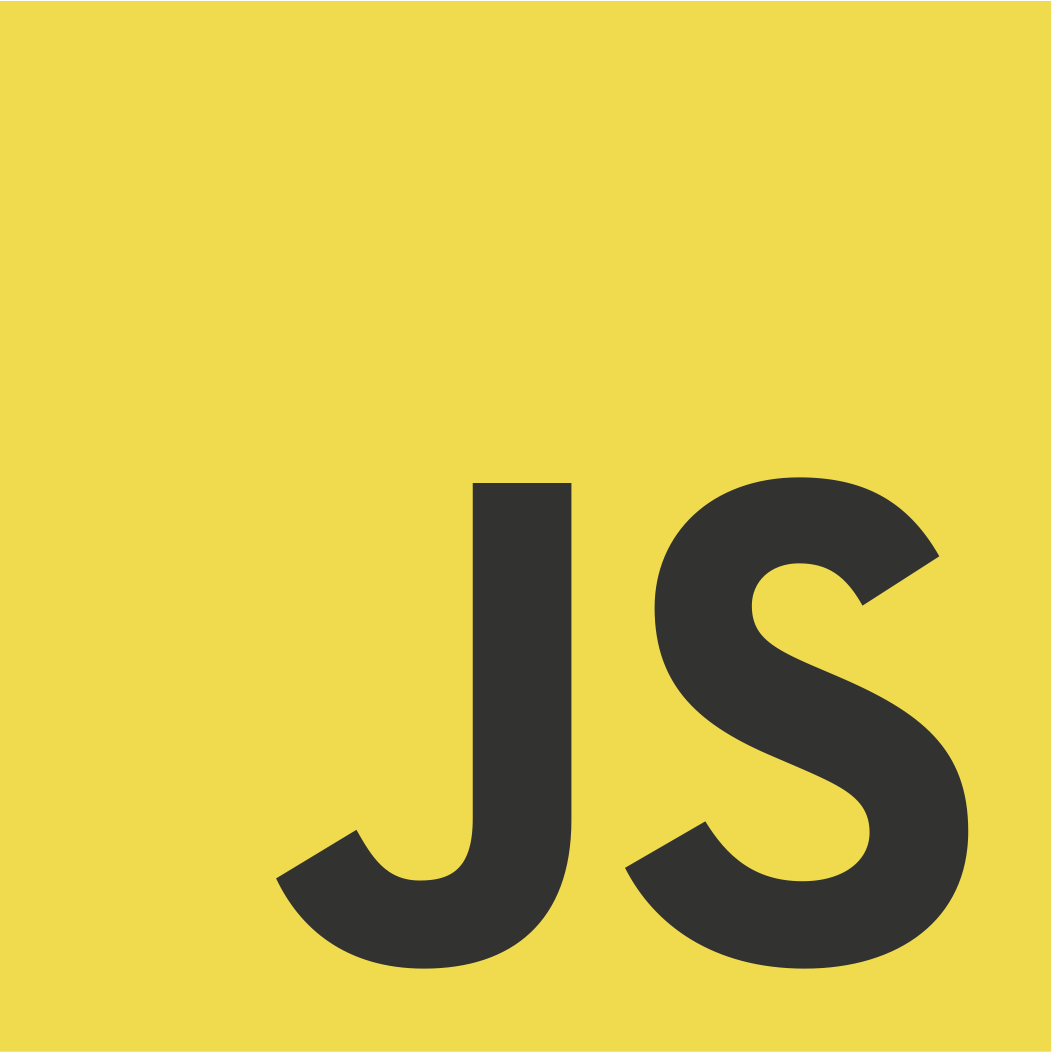
WhatsApp verzendenBericht opnieuw verzendenSynchronisatieBerichten ophalenControlenummerAuthenticatie |
WhatsApp API verzendenDit API-eindpunt wordt gebruikt om WhatsApp-berichten te verzenden. Eindpunt:GET POST https://api.smsmobileapi.com/sendsms
Parameters:
Voorbeeld zonder media:
GET https://api.smsmobileapi.com/sendsms?apikey=YOUR_API_KEY&waonly=yes&recipients=191728660&message=Hello%20World
Voorbeeld met media:
GET https://api.smsmobileapi.com/sendsms?apikey=YOUR_API_KEY&waonly=yes&recipients=191728660&message=Hello%20World&url_media=https://smsmobileapi.com/logo.png
Tekst opmaken in WhatsApp:
Een niet-verzonden WhatsApp-bericht opnieuw verzendenDit API-eindpunt wordt gebruikt om een niet-verzonden bericht opnieuw te verzenden (alleen als het bericht een foutstatus heeft) Eindpunt:Parameter:
Voorbeeld:
KRIJG https://api.smsmobileapi.com/resendwa/?apikey=UW_API_SLEUTEL&guid=GUID_VAN_HET_BERICHT
Synchronisatie om bericht op te halenDit API-eindpunt wordt gebruikt om een verzoek te versturen om WhatsApp-berichten te synchroniseren en op te halen. Eindpunt:GET https://api.smsmobileapi.com/getwa/synchronisation/
Parameter:
Voorbeeld:
GET https://api.smsmobileapi.com/getwa/synchronisation/?apikey=YOUR_API_KEY
Antwoord :
{
WhatsApp-bericht ophalenDit API-eindpunt wordt gebruikt om ontvangen WhatsApp-berichten op te halen. Eindpunt:GET https://api.smsmobileapi.com/getwa
Parameter:
Voorbeeld:
GET https://api.smsmobileapi.com/getwa/?apikey=YOUR_API_KEY
Antwoord :
{
Controleer nummer op WhatsAppMet dit API-eindpunt kunt u controleren of het telefoonnummer beschikbaar is op het WhatsApp-netwerk. Eindpunt:GET https://api.smsmobileapi.com/whatsapp/checknumber/
Parameter:
Voorbeeld:
GET https://api.smsmobileapi.com/whatsapp/checknumber/?recipients=_YOUR_API_KEY_&apikey=_PHONE_NUMBER_
Antwoord :
{
AuthenticatieDe SMSMobile API ondersteunt twee authenticatiemethoden: met een eenvoudige API-sleutel of met het OAuth2-protocol met een client-ID en clientgeheim. 1. API-sleutelauthenticatieOm WhatsApp-berichten via onze API te versturen of op te halen, is authenticatie vereist met behulp van een API-sleutel. Dit garandeert veilige en geautoriseerde toegang tot de berichtenservice. Voer eenvoudig uw API-sleutel in het verzoek in om te authenticeren en naadloos berichten te versturen.2. OAuth2-authenticatieOAuth2 biedt een veiligere en schaalbare authenticatiemethode. Een toegangstoken verkrijgenOm een toegangstoken te verkrijgen, stuurt u een POST-aanvraag naar het tokeneindpunt met uw client-ID en clientgeheim.
curl -X POST https://api.smsmobileapi.com/oauth2/token \
Het toegangstoken gebruiken:Zodra u de toegangstoken hebt, voegt u deze toe aan de Autorisatie header van uw API-verzoeken:
curl -X POST https://api.smsmobileapi.com/sendsms?waonly=yes \
Which Method Should You Use?- Use API Key Authentication for quick and straightforward integrations. - Use OAuth2 Authentication for enhanced security and scalability in your integrations. |
Het is ook mogelijk om stuur tegelijkertijd een WhatsApp-bericht en een sms.
Als u vragen, suggesties of hulp nodig hebt, staat ons team voor u klaar.
Neem gerust contact met ons op via api@smsmobileapi.com